CI workflow
You can use a CI workflow to run end-to-end tests that allow you to continuously check for breakages. We recommend using the following GitHub workflows for every PR in your GitHub repository to run end-to-end tests against a range of Grafana versions.
These are generic examples based on frontend and backend plugins. You may need to alter or remove some of the steps in the playwright-tests
job before using it in your plugin.
Backend plugin workflow
- npm
- yarn
- pnpm
name: E2E tests
on:
pull_request:
permissions:
contents: read
id-token: write
jobs:
resolve-versions:
name: Resolve Grafana images
runs-on: ubuntu-latest
timeout-minutes: 3
outputs:
matrix: ${{ steps.resolve-versions.outputs.matrix }}
steps:
- name: Checkout
uses: actions/checkout@v4
- name: Resolve Grafana E2E versions
id: resolve-versions
uses: grafana/plugin-actions/e2e-version@main
playwright-tests:
needs: resolve-versions
timeout-minutes: 60
strategy:
fail-fast: false
matrix:
GRAFANA_IMAGE: ${{fromJson(needs.resolve-versions.outputs.matrix)}}
name: e2e ${{ matrix.GRAFANA_IMAGE.name }}@${{ matrix.GRAFANA_IMAGE.VERSION }}
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v4
- name: Setup Node.js environment
uses: actions/setup-node@v4
with:
node-version-file: .nvmrc
- name: Install npm dependencies
run: npm ci
- name: Install Mage
uses: magefile/mage-action@v3
with:
install-only: true
- name: Build binaries
run: mage -v build:linux
- name: Build frontend
run: npm run build
- name: Install Playwright Browsers
run: npx playwright install --with-deps
- name: Start Grafana
run: |
docker-compose pull
GRAFANA_VERSION=${{ matrix.GRAFANA_IMAGE.VERSION }} GRAFANA_IMAGE=${{ matrix.GRAFANA_IMAGE.NAME }} docker-compose up -d
- name: Wait for Grafana to start
uses: nev7n/wait_for_response@v1
with:
url: 'http://localhost:3000/'
responseCode: 200
timeout: 60000
interval: 500
- name: Run Playwright tests
id: run-tests
run: npx playwright test
# Uncomment this step to upload the Playwright report to Github artifacts.
# If your repository is public, the report will be public on the Internet so beware not to expose sensitive information.
# - name: Upload artifacts
# uses: actions/upload-artifact@v4
# if: ${{ (always() && steps.run-tests.outcome == 'success') || (failure() && steps.run-tests.outcome == 'failure') }}
# with:
# name: playwright-report-${{ matrix.GRAFANA_IMAGE.NAME }}-v${{ matrix.GRAFANA_IMAGE.VERSION }}-${{github.run_id}}
# path: playwright-report/
# retention-days: 30
name: E2E tests
on:
pull_request:
permissions:
contents: read
id-token: write
jobs:
resolve-versions:
name: Resolve Grafana images
runs-on: ubuntu-latest
timeout-minutes: 3
outputs:
matrix: ${{ steps.resolve-versions.outputs.matrix }}
steps:
- name: Checkout
uses: actions/checkout@v4
- name: Resolve Grafana E2E versions
id: resolve-versions
uses: grafana/plugin-actions/e2e-version@main
playwright-tests:
needs: resolve-versions
timeout-minutes: 60
strategy:
fail-fast: false
matrix:
GRAFANA_IMAGE: ${{fromJson(needs.resolve-versions.outputs.matrix)}}
name: e2e ${{ matrix.GRAFANA_IMAGE.name }}@${{ matrix.GRAFANA_IMAGE.VERSION }}
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v4
- name: Setup Node.js environment
uses: actions/setup-node@v4
with:
node-version-file: .nvmrc
- name: Install yarn dependencies
run: yarn install
- name: Install Mage
uses: magefile/mage-action@v3
with:
install-only: true
- name: Build binaries
run: mage -v build:linux
- name: Build frontend
run: yarn build
- name: Install Playwright Browsers
run: yarn playwright install --with-deps
- name: Start Grafana
run: |
docker-compose pull
GRAFANA_VERSION=${{ matrix.GRAFANA_IMAGE.VERSION }} GRAFANA_IMAGE=${{ matrix.GRAFANA_IMAGE.NAME }} docker-compose up -d
- name: Wait for Grafana to start
uses: nev7n/wait_for_response@v1
with:
url: 'http://localhost:3000/'
responseCode: 200
timeout: 60000
interval: 500
- name: Run Playwright tests
id: run-tests
run: yarn playwright test
# Uncomment this step to upload the Playwright report to Github artifacts.
# If your repository is public, the report will be public on the Internet so beware not to expose sensitive information.
# - name: Upload artifacts
# uses: actions/upload-artifact@v4
# if: ${{ (always() && steps.run-tests.outcome == 'success') || (failure() && steps.run-tests.outcome == 'failure') }}
# with:
# name: playwright-report-${{ matrix.GRAFANA_IMAGE.NAME }}-v${{ matrix.GRAFANA_IMAGE.VERSION }}-${{github.run_id}}
# path: playwright-report/
# retention-days: 30
name: E2E tests
on:
pull_request:
permissions:
contents: read
id-token: write
jobs:
resolve-versions:
name: Resolve Grafana images
runs-on: ubuntu-latest
timeout-minutes: 3
outputs:
matrix: ${{ steps.resolve-versions.outputs.matrix }}
steps:
- name: Checkout
uses: actions/checkout@v4
- name: Resolve Grafana E2E versions
id: resolve-versions
uses: grafana/plugin-actions/e2e-version@main
playwright-tests:
needs: resolve-versions
timeout-minutes: 60
strategy:
fail-fast: false
matrix:
GRAFANA_IMAGE: ${{fromJson(needs.resolve-versions.outputs.matrix)}}
name: e2e ${{ matrix.GRAFANA_IMAGE.name }}@${{ matrix.GRAFANA_IMAGE.VERSION }}
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v4
- uses: pnpm/action-setup@v3
- name: Setup Node.js environment
uses: actions/setup-node@v4
with:
node-version-file: .nvmrc
- name: Install pnpm dependencies
run: pnpm install --frozen-lockfile
- name: Install Mage
uses: magefile/mage-action@v3
with:
install-only: true
- name: Build binaries
run: mage -v build:linux
- name: Build frontend
run: pnpm run build
- name: Install Playwright Browsers
run: pnpm playwright install --with-deps
- name: Start Grafana
run: |
docker-compose pull
GRAFANA_VERSION=${{ matrix.GRAFANA_IMAGE.VERSION }} GRAFANA_IMAGE=${{ matrix.GRAFANA_IMAGE.NAME }} docker-compose up -d
- name: Wait for Grafana to start
uses: nev7n/wait_for_response@v1
with:
url: 'http://localhost:3000/'
responseCode: 200
timeout: 60000
interval: 500
- name: Run Playwright tests
id: run-tests
run: pnpm playwright test
# Uncomment this step to upload the Playwright report to Github artifacts.
# If your repository is public, the report will be public on the Internet so beware not to expose sensitive information.
# - name: Upload artifacts
# uses: actions/upload-artifact@v4
# if: ${{(always() && steps.run-tests.outcome == 'success') || (failure() && steps.run-tests.outcome == 'failure') }}
# with:
# name: playwright-report-${{ matrix.GRAFANA_IMAGE.NAME }}-v${{ matrix.GRAFANA_IMAGE.VERSION }}-${{github.run_id}}
# path: playwright-report/
# retention-days: 30
Frontend plugin workflow
- npm
- yarn
- pnpm
name: E2E tests
on:
pull_request:
permissions:
contents: read
id-token: write
jobs:
resolve-versions:
name: Resolve Grafana images
runs-on: ubuntu-latest
timeout-minutes: 3
outputs:
matrix: ${{ steps.resolve-versions.outputs.matrix }}
steps:
- name: Checkout
uses: actions/checkout@v4
- name: Resolve Grafana E2E versions
id: resolve-versions
uses: grafana/plugin-actions/e2e-version@main
playwright-tests:
needs: resolve-versions
timeout-minutes: 60
strategy:
fail-fast: false
matrix:
GRAFANA_IMAGE: ${{fromJson(needs.resolve-versions.outputs.matrix)}}
name: e2e ${{ matrix.GRAFANA_IMAGE.name }}@${{ matrix.GRAFANA_IMAGE.VERSION }}
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v4
- name: Setup Node.js environment
uses: actions/setup-node@v4
with:
node-version-file: .nvmrc
- name: Install npm dependencies
run: npm ci
- name: Build frontend
run: npm run build
- name: Install Playwright Browsers
run: npx playwright install --with-deps
- name: Start Grafana
run: |
docker-compose pull
GRAFANA_VERSION=${{ matrix.GRAFANA_IMAGE.VERSION }} GRAFANA_IMAGE=${{ matrix.GRAFANA_IMAGE.NAME }} docker-compose up -d
- name: Wait for Grafana to start
uses: nev7n/wait_for_response@v1
with:
url: 'http://localhost:3000/'
responseCode: 200
timeout: 60000
interval: 500
- name: Run Playwright tests
id: run-tests
run: npx playwright test
# Uncomment this step to upload the Playwright report to Github artifacts.
# If your repository is public, the report will be public on the Internet so beware not to expose sensitive information.
# - name: Upload artifacts
# uses: actions/upload-artifact@v4
# if: ${{ (always() && steps.run-tests.outcome == 'success') || (failure() && steps.run-tests.outcome == 'failure') }}
# with:
# name: playwright-report-${{ matrix.GRAFANA_IMAGE.NAME }}-v${{ matrix.GRAFANA_IMAGE.VERSION }}-${{github.run_id}}
# path: playwright-report/
# retention-days: 30
name: E2E tests
on:
pull_request:
permissions:
contents: read
id-token: write
jobs:
resolve-versions:
name: Resolve Grafana images
runs-on: ubuntu-latest
timeout-minutes: 3
outputs:
matrix: ${{ steps.resolve-versions.outputs.matrix }}
steps:
- name: Checkout
uses: actions/checkout@v4
- name: Resolve Grafana E2E versions
id: resolve-versions
uses: grafana/plugin-actions/e2e-version@main
playwright-tests:
needs: resolve-versions
timeout-minutes: 60
strategy:
fail-fast: false
matrix:
GRAFANA_IMAGE: ${{fromJson(needs.resolve-versions.outputs.matrix)}}
name: e2e ${{ matrix.GRAFANA_IMAGE.name }}@${{ matrix.GRAFANA_IMAGE.VERSION }}
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v4
- name: Setup Node.js environment
uses: actions/setup-node@v4
with:
node-version-file: .nvmrc
- name: Install yarn dependencies
run: yarn install
- name: Build frontend
run: yarn build
- name: Install Playwright Browsers
run: yarn playwright install --with-deps
- name: Start Grafana
run: |
docker-compose pull
GRAFANA_VERSION=${{ matrix.GRAFANA_IMAGE.VERSION }} GRAFANA_IMAGE=${{ matrix.GRAFANA_IMAGE.NAME }} docker-compose up -d
- name: Wait for Grafana to start
uses: nev7n/wait_for_response@v1
with:
url: 'http://localhost:3000/'
responseCode: 200
timeout: 60000
interval: 500
- name: Run Playwright tests
id: run-tests
run: yarn playwright test
# Uncomment this step to upload the Playwright report to Github artifacts.
# If your repository is public, the report will be public on the Internet so beware not to expose sensitive information.
# - name: Upload artifacts
# uses: actions/upload-artifact@v4
# if: ${{ (always() && steps.run-tests.outcome == 'success') || (failure() && steps.run-tests.outcome == 'failure') }}
# with:
# name: playwright-report-${{ matrix.GRAFANA_IMAGE.NAME }}-v${{ matrix.GRAFANA_IMAGE.VERSION }}-${{github.run_id}}
# path: playwright-report/
# retention-days: 30
name: E2E tests
on:
pull_request:
permissions:
contents: read
id-token: write
jobs:
resolve-versions:
name: Resolve Grafana images
runs-on: ubuntu-latest
timeout-minutes: 3
outputs:
matrix: ${{ steps.resolve-versions.outputs.matrix }}
steps:
- name: Checkout
uses: actions/checkout@v4
- name: Resolve Grafana E2E versions
id: resolve-versions
uses: grafana/plugin-actions/e2e-version@main
playwright-tests:
needs: resolve-versions
timeout-minutes: 60
strategy:
fail-fast: false
matrix:
GRAFANA_IMAGE: ${{fromJson(needs.resolve-versions.outputs.matrix)}}
name: e2e ${{ matrix.GRAFANA_IMAGE.name }}@${{ matrix.GRAFANA_IMAGE.VERSION }}
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v4
- uses: pnpm/action-setup@v3
- name: Setup Node.js environment
uses: actions/setup-node@v4
with:
node-version-file: .nvmrc
- name: Install pnpm dependencies
run: pnpm install --frozen-lockfile
- name: Build frontend
run: pnpm run build
- name: Install Playwright Browsers
run: pnpm playwright install --with-deps
- name: Start Grafana
run: |
docker-compose pull
GRAFANA_VERSION=${{ matrix.GRAFANA_IMAGE.VERSION }} GRAFANA_IMAGE=${{ matrix.GRAFANA_IMAGE.NAME }} docker-compose up -d
- name: Wait for Grafana to start
uses: nev7n/wait_for_response@v1
with:
url: 'http://localhost:3000/'
responseCode: 200
timeout: 60000
interval: 500
- name: Run Playwright tests
id: run-tests
run: pnpm playwright test
# Uncomment this step to upload the Playwright report to Github artifacts.
# If your repository is public, the report will be public on the Internet so beware not to expose sensitive information.
# - name: Upload artifacts
# uses: actions/upload-artifact@v4
# if: ${{ (always() && steps.run-tests.outcome == 'success') || (failure() && steps.run-tests.outcome == 'failure') }}
# with:
# name: playwright-report-${{ matrix.GRAFANA_IMAGE.NAME }}-v${{ matrix.GRAFANA_IMAGE.VERSION }}-${{github.run_id}}
# path: playwright-report/
# retention-days: 30
The e2e-versions Action
These example workflows have a job called Resolve Grafana images
that uses the e2e-version Action to resolve a list of Grafana images. For every image returned, a new job is started that builds the plugin, starts Grafana, and runs the end-to-end tests.
The Action supports two modes:
plugin-grafana-dependency
version-support-policy
.
Use the plugin-grafana-dependency mode
The plugin-grafana-dependency
mode is the default, so if you don't specify a value for the version-resolver-type
input parameter, this is the resolver that will be used.
This mode returns the most recent grafana-dev image. Additionally, it returns all the latest patch releases of Grafana Enterprise since the version that was specified as grafanaDependency
in the plugin.json. To avoid starting too many jobs, the output is capped at 6 versions.
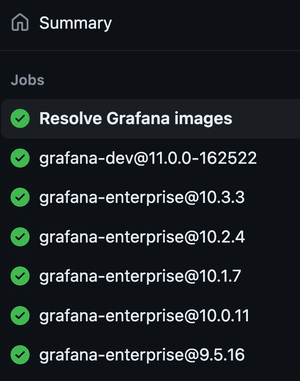
Use the version-support-policy mode
Except for resolving the most recent grafana-dev
image, the version-support-policy
mode resolves versions according to Grafana's plugin compatibility support policy. Specifically, it retrieves the latest patch release for each minor version within the current major version of Grafana. Additionally, it includes the most recent release for the latest minor version of the previous major Grafana version.
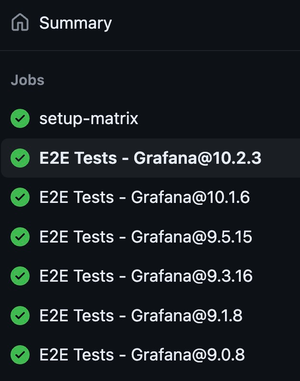
To use the version-support-policy
mode, you need to specify the version-resolver-type
input argument like in this example:
- name: Resolve Grafana E2E versions
id: resolve-versions
uses: grafana/plugin-actions/e2e-version
with:
version-resolver-type: version-support-policy
Playwright report
The end-to-end tooling generates a Playwright HTML test report for every Grafana version that is being tested. In case any of the tests fail, a Playwright trace viewer is also generated along with the report. The Upload artifacts
step in the example workflows uploads the report to GitHub as an artifact.
To find information on how to download and view the report, refer to the Playwright documentation.