Important: This documentation is about an older version. It's relevant only to the release noted, many of the features and functions have been updated or replaced. Please view the current version.
MongoDB query editor
Grafana provides a query editor for MongoDB that supports the same syntax as the MongoDB Shell (mongosh), but with some limitations:
- You can only run one command or query per query
- Only
find
andaggregate
read commands are supported ISODate
is the only supported object constructor
For an introduction to writing scripts for the MongoDB shell see Write scripts in MongoDB’s documentation. You create a query in Grafana’s query editor in the same way you would in the MongoDB shell.
Example:
sample_mflix.movies.aggregate([
{"$match": { "year": {"$gt" : 2000} }},
{"$group": { "_id": "$year", "count": { "$sum": 1 }}},
{"$project": { "_id": 0, "count": 1, "time": { "$dateFromParts": {"year": "$_id", "month": 2}}}}
]
).sort({"time": 1})
Additional syntax
The editor extends the MongoDB Shell syntax by means of database selection, where you can use a database name instead of db
. For example, sample_mflix.movies.find()
. You can still use db
to refer to the default database in your connection string.
It also extends it by means of aggregate sorting. Sorting typically happens within the aggregate pipeline. The extended syntax is allowed on aggregate
similarly to find
. For example, sample_mflix.movies.aggregate({}).sort({"time": 1})
.
Note
MongoDB does not perform the sort with this syntax. The sort happens after the results are queried from the collection.
Collections with a dot
For collections containing a dot you can use the following syntax:
my_db.getCollection("my.collection").find({})
Keyboard shortcuts
Press Ctrl + Space to show code completion, which is displayed after entering a .
after a database, collection, query method, or aggregation method name.
Cmd + S runs the query.
Query as time series
Make a time series query by aliasing a date field to time
.
Note
You can coerce non-date fields into date fields and alias them totime
to use them to make a time series query.
The following query converts the int
field year
to a date that is projected as time
using the MongoDB $dateFromParts pipeline operator:
sample_mflix.movies.aggregate([
{"$match": { "year": {"$gt" : 2000} }},
{"$group": { "_id": "$year", "count": { "$sum": 1 }}},
{"$project": { "_id": 0, "count": 1, "time": { "$dateFromParts": {"year": "$_id", "month": 2}}}}
]
).sort({"time": 1})
If you want to group your time series by Metric, project a field called __metric
.
The following query displays the count of movies over time by movie rating using __metric
:
sample_mflix.movies.aggregate([
{"$match": { "year": {"$gt" : 2000}}},
{"$group": { "_id": {"year":"$year", "rated":"$rated"}, "count": { "$sum": 1 } }},
{"$project": { "_id": 0, "time": { "$dateFromParts": {"year": "$_id.year", "month": 2}}, "__metric": "$_id.rated", "count": 1}}
]
).sort({"time": 1})
Diagnostics
For information about diagnostics commands, see Diagnostic Commands.
This plugin supports the following MongoDB diagnostic commands:
buildInfo
connPoolStats
connectionStatus
dbStats
getLog
hostInfo
lockInfo
replSetGetStatus
serverStatus
stats
Macros
To simplify syntax and to allow for dynamic times, you can write queries that contain macros.
- $__timeFrom
- Will be replaced by the start of the currently active time selection converted to
time
data type. - $__timeTo
- Will be replaced by the end of the currently active time selection converted to
time
data type.
Was this page helpful?
Related resources from Grafana Labs


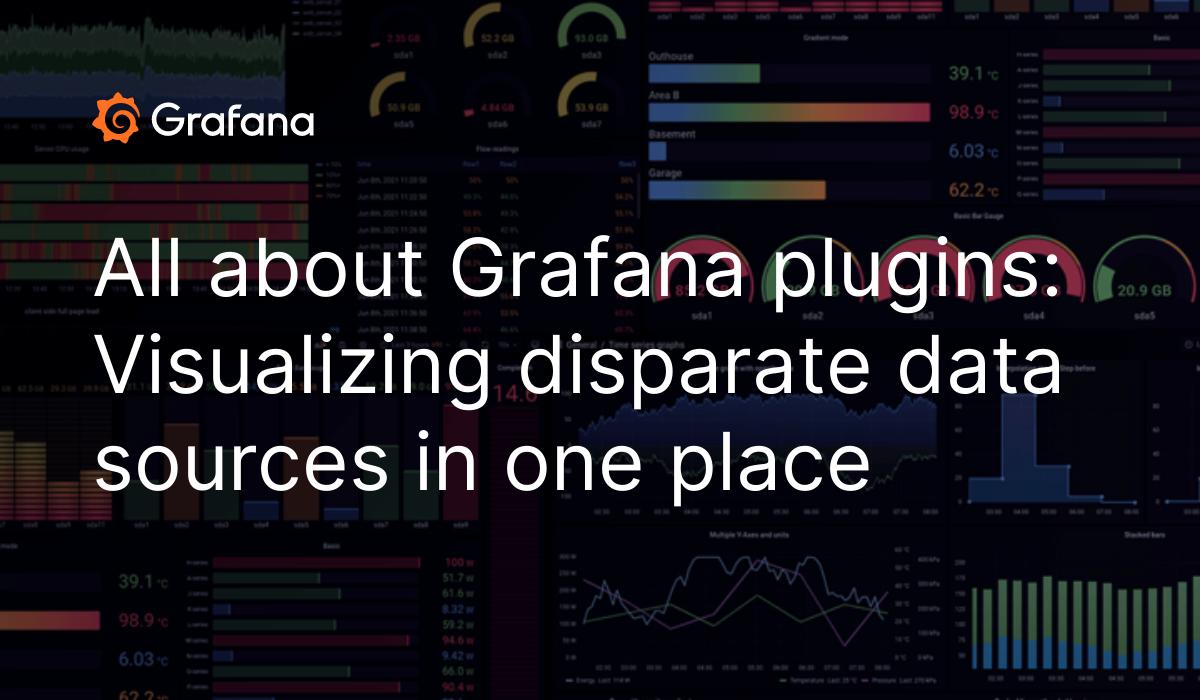